This post will demonstrate how to push data into Power BI Streaming Datasets with C#. For demo purposes I normally use LINQPad to run the code, but you could also create a .Net or .Net Core console application. LINQPad is an excellent, lightweight scratchpad for C# and other .Net languages.
Power BI Streaming Datasets are a very cool feature because dashboard tiles that use them update in real time. You don’t have to refresh the browser window to display new data. With this feature you can watch your data in near real-time. This could be compelling in scenarios involving sensors, IoT, website traffic, etc. Here is an example of streaming dataset tiles:

Create the Dataset
From the home page of a Power BI workspace, click Create in the top right corner.
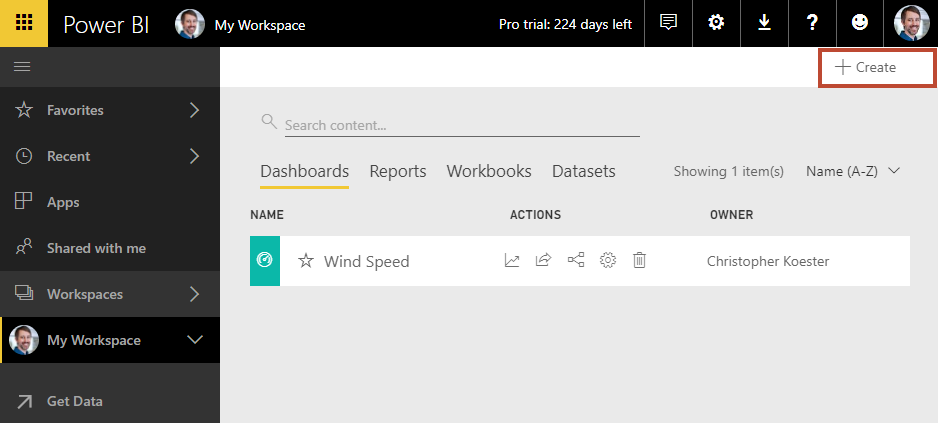
In the dropdown, select Streaming dataset.
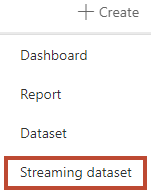
In the New streaming dataset window, select API and then Next.
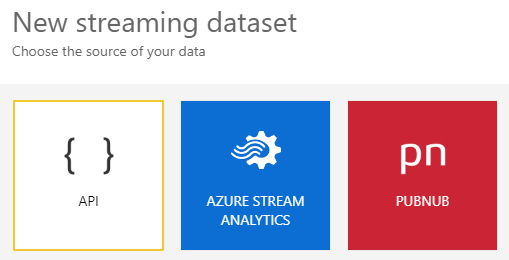
Enter a name for the dataset and add values as shown below. As you can see from the values, this demo simulates wind speed data from a weather station.
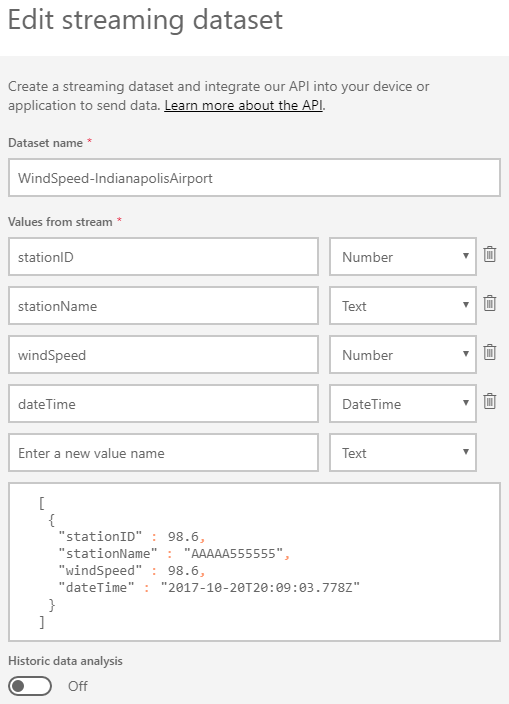
When done, select Create to create the dataset. Once the dataset is created, copy the Push URL that appears and save it somewhere.
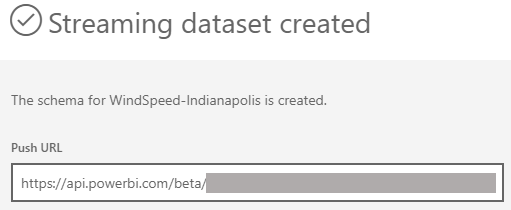
Dashboard
Now that the dataset has been created, it’s time to add a dashboard tile. Navigate to a dashboard where you want to add the tile. Click Add tile and then select CUSTOM STREAMING DATA.
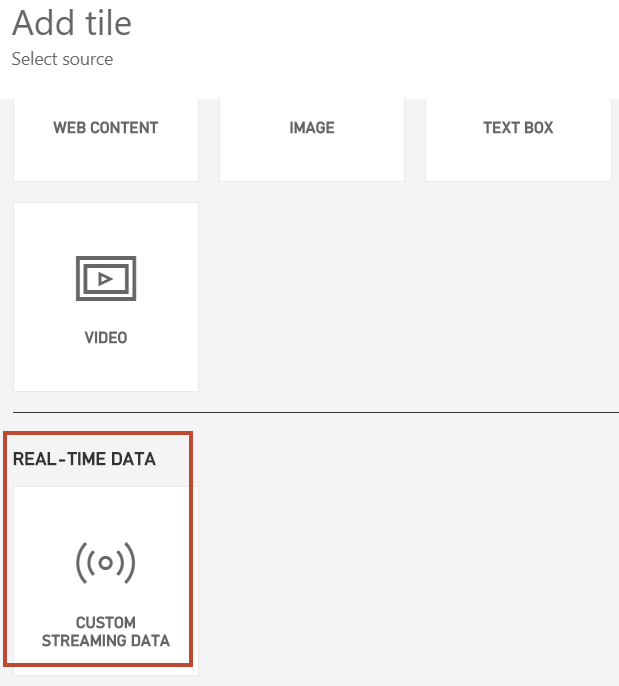
Select the dataset that you previously created and click Next.
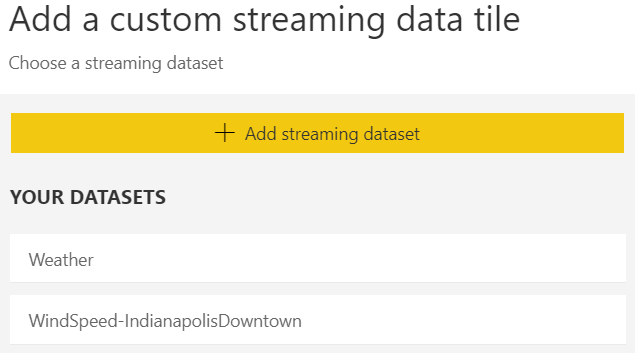
On the Tile details pane, enter the values as shown and click Next. On the next pane, enter a title or subtitle if you wish and then click Apply.
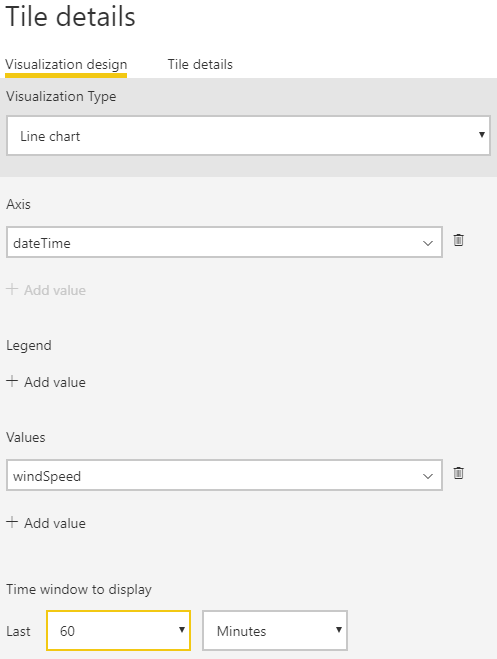
Adjust the tile as desired. The size shown below works well.
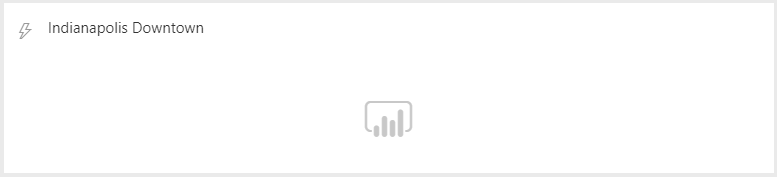
C# Code
The Power BI dataset and dashboard should now be ready for streaming data. The code below will push random/demo data into the previously created streaming dataset with a duration and frequency of your choosing. It was written for demonstration purposes, and as I previously mentioned, I normally run it in LINQPad.
The following namespaces are used, with Json.NET 10.0.3 installed via NuGet. If you’re using a LINQPad version less than Developer, you’ll need to download the Json.NET assemblies and reference them manually.
/*
Generates random wind speed observations and pushes them to a Power BI streaming dataset.
Supply your own values in the Main method on line 24 - most importantly the Power BI push URL. The script will not run without it.
On lines 19-20 you can set the script duration and data push frequency.
*/
using Newtonsoft.Json
using System.Net.Http
using System.Threading.Tasks
class Program
{
// Use class-level Random for best results
static Random random = new Random();
// Use class-level HttpClient as a best practice
static HttpClient client = new HttpClient();
static List<WeatherStation> weatherStation;
static void Main()
{
const int duration = 60; // Length of time in minutes to push data
const int pauseInterval = 2; // Frequency in seconds that data will be pushed
const string timeFormat = "yyyy-MM-ddTHH:mm:ss.fffZ"; // Time format required by Power BI
weatherStation = new List<WeatherStation>();
weatherStation.Add(new WeatherStation { stationID = 1, stationName = "<Station Name>", powerBiPostUrl = "<Power BI push (Post) URL>" });
GenerateObservations(duration, pauseInterval, timeFormat);
}
public static void GenerateObservations(int duration, int pauseInterval, string timeFormat)
{
DateTime startTime = GetDateTimeUtc();
DateTime currentTime;
do
{
foreach (var station in weatherStation)
{
WindSpeedRecording windSpeedRecording = new WindSpeedRecording
{
stationID = station.stationID,
stationName = station.stationName,
windSpeed = GenerateRandom(10),
dateTime = GetDateTimeUtc().ToString(timeFormat)
};
var jsonString = JsonConvert.SerializeObject(windSpeedRecording);
var postToPowerBi = HttpPostAsync(station.powerBiPostUrl, "[" + jsonString + "]"); // Add brackets for Power BI
Console.WriteLine(jsonString);
}
Thread.Sleep(pauseInterval * 1000); // Pause for n seconds. Not highly accurate.
currentTime = GetDateTimeUtc();
} while ((currentTime - startTime).TotalMinutes <= duration);
}
public class WindSpeedRecording
{
public int stationID { get; set; }
public string stationName { get; set; }
public int windSpeed { get; set; }
public string dateTime { get; set; }
}
public class WeatherStation
{
public int stationID { get; set; }
public string stationName { get; set; }
public string powerBiPostUrl { get; set; }
}
static int GenerateRandom(int max)
{
int n = random.Next(max);
return n;
}
static async Task<HttpResponseMessage> HttpPostAsync(string url, string data)
{
// Construct an HttpContent object from StringContent
HttpContent content = new StringContent(data);
HttpResponseMessage response = await client.PostAsync(url, content);
response.EnsureSuccessStatusCode();
return response;
}
static DateTime GetDateTimeUtc()
{
return DateTime.UtcNow;
}
}
While the script is running, the streaming dataset tile should update in real time.

Reference
Power BI Service Real Time Streaming
Power BI Streaming Datasets Hands-on Lab
Linked is a similar tutorial that also highlights PowerShell and Flow. My post differs by focusing on a C# script that can be run on demand in LINQPad, with an easily changeable duration and push interval. The code above should also be compatible with .NET Core.
Integrate Power BI Into Your Application: Part 6 – Real-time Streaming and Push Data
Similar tutorial by Reza Rad that makes use of the Power BI REST API. My post differs by pushing directly to a Streaming Dataset push URL instead of using the REST API.